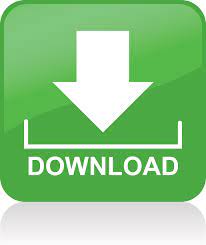

Each node has a value and a link to next node. However, by and large, books are not very helpful because what you need is deliberate practice not reading books. Elements of Programming Interviews is better. A characteristic specific to linked lists is that the order of the nodes isn. Linked Lists are a data structure that represents a linear collection of nodes. These articles go over different kinds of Algorithmic Thinking.All are based on LeetCode problems. The coding questions there are obsolete and too easy. If youre interested in reading more about Programming Interview Questions in general, weve compiled a lengthy list of these questions, including their explanations, implementations, visual representations and applications.
#Elements of programming interviews vs leetcode how to
The implementation of a linked list is pretty simple in Java. How to crack the coding interview throw your book Cracking the Coding Interview into the trash. System.arraycopy(Object src, int srcPos, Object dest, int destPos, int length)ġ) Rotate Array, Reverse Words in a StringĢ) Evaluate Reverse Polish Notation (Stack)Ĥ) Word Ladder (BFS), Word Ladder II (BFS)Ħ) Wildcard Matching, Regular Expression Matchingĩ) Two Sum, Two Sum II, Two Sum III, 3Sum, 4SumĢ5) Remove Duplicates from Sorted Array: I, II, Remove Element, Move ZeroesĢ7) Longest Substring Without Repeating CharactersĢ8) Longest Substring that contains 2 unique characters Ģ8) Substring with Concatenation of All Wordsģ1) Find Minimum in Rotated Sorted Array: I, IIĤ8) Basic Calculator, Basic Calculator IIĥ4) Get Target Using Number List And Arithmetic Operationsĥ7) Missing Number, Find the duplicate number, First Missing Positiveħ0) Intersection of Two Arrays, Intersection of Two Arrays IIħ1) Sliding Window Maximum, Moving Average from Data StreamĬommon methods to solve matrix related problem include DFS, BFS, dynamic programming, etc.Ĩ) Number of Islands (DFS/BFS), Number of Islands II (Disjoint Set), Number of Connected Components in an Undirected Graphġ4) Longest Increasing Path in a Matrix (DFS) ToCharArray() //get char array of a StringĬharAt(int x) //get a char at the specific indexĪrrays.toString(char a) //convert to stringĪpyOf(T original, int newLength)
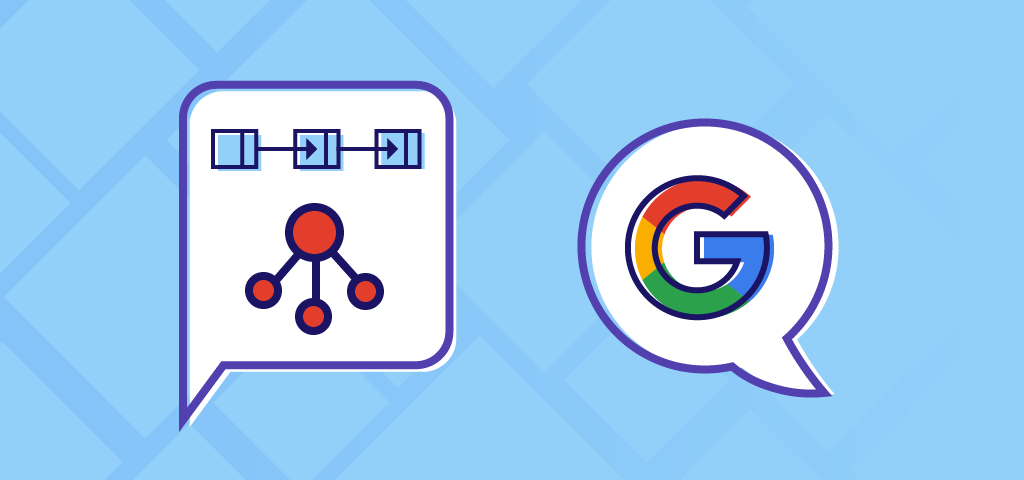
arraycopy ( Object src, int srcPos, Object dest, int destPos, int length ) copyOf (T original, int newLength ) System. toString ( char a ) //convert to string Arrays. you should use two indexes i and j The solution at index i is usually the best that you get by moving the index j between 0 and i. Substring ( int beginIndex, int endIndex ) Integer. How to learn to solve coding interview questions using Leetcode (Part II). ToCharArray ( ) //get char array of a StringĬharAt ( int x ) //get a char at the specific index
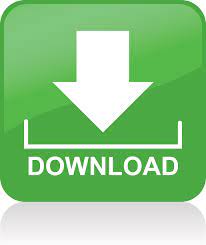